In the fast-paced world of modern software development, concurrency has become a crucial element in creating high-performance applications. As developers, we’re constantly seeking ways to make our code more efficient, responsive, and scalable. Enter virtual threads – the game-changers in the realm of concurrent programming.
But like any powerful tool, they come with their own set of challenges. In this comprehensive guide, we’ll dive deep into the world of virtual threads, exploring how to harness their power while skillfully navigating the potential pitfalls that lie in wait.
Understanding Virtual Threads: The Ninjas of Concurrent Programming
The Basics of Virtual Threads
Virtual threads are the new kids on the block in the world of concurrency, and they’re making quite a splash. Think of them as the ninjas of the programming world – stealthy, efficient, and capable of accomplishing tasks without leaving a trace. Unlike their bulkier cousins, traditional threads, virtual threads are lightweight and nimble, allowing developers to create thousands or even millions of them without breaking a sweat.
But what exactly are virtual threads? At their core, they’re a way to execute concurrent tasks without the overhead typically associated with traditional threads. They’re managed by the Java runtime, which means you don’t have to worry about the nitty-gritty details of thread management. It’s like having a personal assistant who handles all the scheduling and coordination for you.
The key difference between virtual threads and traditional threads lies in their relationship with the operating system. Traditional threads are directly mapped to OS threads, which can be resource-intensive. Virtual threads, on the other hand, are multiplexed onto a smaller number of OS threads, allowing for much greater scalability and efficiency.
Benefits of Virtual Threads
Now that we’ve got the basics down, let’s talk about why virtual threads are causing such a stir in the development community. The benefits are numerous and significant:
- Improved Performance: Virtual threads can significantly boost your application’s performance, especially in scenarios involving I/O-bound operations. It’s like giving your code a triple espresso shot – suddenly, everything moves faster and more efficiently.
- Resource Efficiency: With virtual threads, you can create thousands or even millions of concurrent tasks without exhausting your system resources. It’s akin to fitting an entire circus in a mini cooper – seemingly impossible, yet virtual threads make it happen.
- Scalability: As your application grows, virtual threads grow with it. They provide a level of scalability that was previously difficult to achieve, allowing your application to handle increased load with grace and ease.
- Simplified Programming Model: Virtual threads make concurrent programming more accessible. They abstract away many of the complexities associated with traditional thread management, allowing developers to focus on the business logic rather than the intricacies of thread coordination.
The Importance of Concurrency in Modern Applications
In today’s digital landscape, concurrency isn’t just a nice-to-have feature – it’s a necessity. Modern applications need to handle multiple tasks simultaneously to provide the responsive, efficient experience that users expect. Whether it’s a web server handling thousands of requests per second, a financial system processing high-frequency trades, or a machine learning algorithm crunching vast amounts of data, concurrency is at the heart of it all.
Consider these scenarios:
- A popular e-commerce platform during a flash sale
- A social media app processing millions of user interactions in real-time
- A video streaming service delivering content to users across the globe
All these applications rely heavily on concurrent processing to deliver a smooth, responsive user experience. Without effective concurrency, these applications would crumble under the weight of user demand.
However, implementing concurrency has traditionally been a complex and error-prone task. Developers have long grappled with issues like race conditions, deadlocks, and resource contention. It’s been like trying to choreograph a complex dance routine with blindfolded dancers – challenging, to say the least. This is where virtual threads come in, offering a more manageable approach to concurrent programming.
Embracing Concurrency with Virtual Threads
Efficient Task Management
One of the standout features of virtual threads is their ability to make task management a breeze. With virtual threads, you can create a separate thread for each task, no matter how small, without worrying about the overhead. It’s like having an army of mini-yous, each capable of handling a specific task independently.
Here’s a simple example to illustrate this:
javaCopyvoid processOrders(List<Order> orders) {
orders.forEach(order -> Thread.startVirtualThread(() -> processOrder(order)));
}
In this code snippet, we’re creating a new virtual thread for each order. This level of granularity would be impractical with traditional threads due to the overhead involved. But with virtual threads, it’s not only possible but efficient.
Simplified Programming Model
Virtual threads also simplify the programming model for concurrent applications. They allow you to write code in a more sequential, easy-to-understand manner, even when dealing with concurrent operations. This is particularly beneficial when dealing with blocking I/O operations.
Consider this example:
javaCopyvoid fetchDataFromMultipleSources() {
Thread.startVirtualThread(() -> {
var data1 = fetchFromSource1(); // Blocking I/O
var data2 = fetchFromSource2(); // Blocking I/O
processData(data1, data2);
});
}
In this code, even though we’re performing multiple blocking I/O operations, we don’t need to worry about manually managing thread pools or callback hell. The virtual thread takes care of the concurrency aspects, allowing us to write code that looks and feels sequential.
Common Pitfalls in Virtual Thread Usage
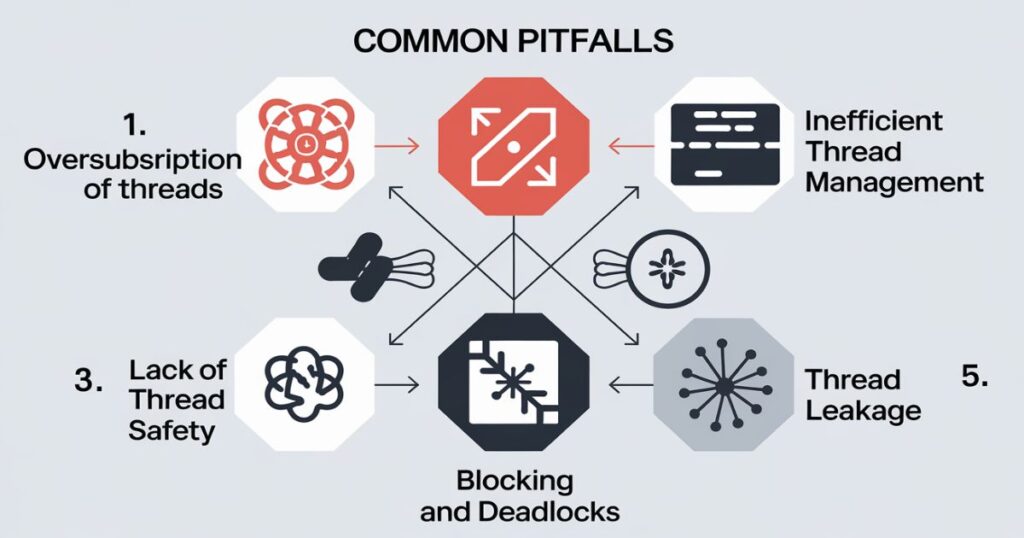
While virtual threads offer numerous benefits, they’re not without their challenges. Like any powerful tool, they need to be used wisely to avoid potential pitfalls. Let’s explore some of the common issues developers might encounter when working with virtual threads.
Overusing Virtual Threads
It’s easy to get carried away with the “create a thread for everything” approach when using virtual threads. After all, they’re lightweight and efficient, right? While it’s true that virtual threads have much less overhead compared to traditional threads, creating too many of them can still lead to performance issues.
Think of it like this: just because you can fit a hundred people in an elevator doesn’t mean you should. At some point, it becomes counterproductive. The same principle applies to virtual threads. Creating a new thread for every tiny task can lead to increased context switching and memory usage, potentially negating the performance benefits.
To avoid this pitfall, consider using thread pools or other concurrency control mechanisms to manage the number of active threads. It’s about finding the right balance for your specific application needs.
Debugging and Monitoring Challenges
Debugging concurrent code has always been challenging, and virtual threads add another layer of complexity to this task. When you have thousands of virtual threads running simultaneously, pinpointing the source of an issue can feel like playing Where’s Waldo on expert mode.
Traditional debugging techniques may not be as effective when dealing with virtual threads. Stack traces, for instance, might not provide the full picture as they do with traditional threads. This is because virtual threads can be suspended and resumed, potentially across different carrier threads.
To mitigate these challenges:
- Use specialized debugging tools that are aware of virtual threads.
- Implement comprehensive logging and monitoring solutions.
- Develop a solid understanding of how virtual threads operate under the hood.
Resource Management
While virtual threads are more resource-efficient than traditional threads, they’re not magic. They still consume system resources, and improper management can lead to issues like memory leaks or resource exhaustion.
It’s crucial to implement proper resource management strategies when working with virtual threads. This includes:
- Ensuring that threads are properly terminated when they’re no longer needed.
- Monitoring thread lifecycle and resource usage.
- Implementing appropriate error handling and recovery mechanisms.
Remember, playing nice with system resources is key to maintaining a healthy, performant application.
Also Read: Unveiling the Symbolism and Design of the Passages Malibu Logo: A Deep Dive
Avoiding the Pitfalls of Virtual Threads
Now that we’ve identified some of the common pitfalls, let’s explore strategies to avoid them and make the most of virtual threads.
1. Overhead Management
To keep your virtual threads lean and mean, consider these strategies:
- Use Thread Pools: Even with virtual threads, using thread pools can help manage concurrency levels and prevent excessive thread creation.
- Batch Operations: When dealing with a large number of small tasks, consider batching them to reduce the number of threads created.
- Monitor Thread Creation: Use monitoring tools to keep an eye on thread creation rates and identify any potential issues.
2. Synchronization Challenges
Synchronization in concurrent programming is like choreographing a ballet with blindfolded dancers – it requires careful planning and execution. With virtual threads, some traditional synchronization techniques may not be as effective or efficient.
To address synchronization challenges:
- Use Thread-Safe Data Structures: Utilize concurrent collections and other thread-safe data structures provided by the Java standard library.
- Minimize Shared State: Where possible, design your application to minimize shared mutable state between threads.
- Consider Lock-Free Algorithms: For high-performance scenarios, explore lock-free and wait-free algorithms that can reduce contention between threads.
3. Deadlock Prevention
Deadlocks are the bane of concurrent programming, and they can still occur with virtual threads. It’s like a Mexican standoff, but with code – nobody wins, and your application grinds to a halt.
To prevent deadlocks:
- Order Lock Acquisition: Always acquire locks in a consistent order across your application.
- Use Timeouts: Implement timeouts when acquiring locks to prevent indefinite waiting.
- Avoid Nested Locks: Minimize the use of nested locks, as they increase the complexity of your code and the likelihood of deadlocks.
4. Thread Lifecycle Management
Proper management of thread lifecycles is crucial for maintaining a healthy application. This involves creating threads when needed, monitoring their execution, and ensuring they’re properly terminated when no longer required.
Best practices for thread lifecycle management include:
- Use Structured Concurrency: Leverage structured concurrency constructs to ensure that child tasks complete before their parent tasks.
- Implement Proper Error Handling: Ensure that exceptions in virtual threads are properly caught and handled to prevent resource leaks.
- Monitor Thread Lifespan: Use monitoring tools to track the lifespan of your virtual threads and identify any long-running or stuck threads.
5. Balancing Task Granularity
Finding the right balance in task granularity is crucial for optimal performance. Tasks that are too small can lead to excessive overhead, while tasks that are too large can reduce concurrency.
To achieve the right balance:
- Profile Your Application: Use profiling tools to understand the performance characteristics of your tasks.
- Experiment with Different Granularities: Test different task sizes to find the optimal balance for your specific use case.
- Consider Adaptive Approaches: Implement adaptive algorithms that can adjust task granularity based on runtime conditions.
Strategies for Avoiding Pitfalls
Balancing Concurrency Levels
Finding the right level of concurrency for your application is like tuning a guitar – it requires careful adjustment to get the perfect sound. Too little concurrency, and you’re not taking full advantage of your resources. Too much, and you risk overwhelming your system.
To find the sweet spot:
- Start Conservative: Begin with a conservative number of threads and gradually increase as needed.
- Monitor System Resources: Keep a close eye on CPU usage, memory consumption, and I/O throughput as you adjust concurrency levels.
- Use Concurrency Profiling Tools: Leverage tools like Java Flight Recorder to gain insights into your application’s concurrency behavior.
Using Profiling Tools
Profiling tools are your Swiss Army knife for performance optimization. They provide valuable insights into how your application behaves under different conditions.
Popular profiling tools for Java applications include:
- Java Flight Recorder (JFR)
- VisualVM
- YourKit Java Profiler
When using these tools:
- Focus on Hot Spots: Identify the areas of your code that consume the most resources or time.
- Analyze Thread Behavior: Look for patterns in thread creation, execution, and termination.
- Compare Before and After: Always benchmark your application before and after making changes to ensure your optimizations are effective.
Implementing Proper Resource Management
Efficient resource management is key to preventing your system from crying under the weight of concurrent operations. Here are some strategies to keep your resources in check:
- Use Resource Pools: Implement object pools for frequently used resources to reduce allocation and deallocation overhead.
- Implement Proper Cleanup: Ensure that resources are properly released when they’re no longer needed, especially in error scenarios.
- Monitor Resource Usage: Use monitoring tools to track resource consumption and identify any leaks or inefficiencies.
Best Practices for Embracing Virtual Threads
1. Profiling and Benchmarking
Before diving headfirst into the world of virtual threads, it’s crucial to establish a baseline for your application’s performance. This allows you to accurately measure the impact of your changes and ensure that you’re moving in the right direction.
Steps for effective profiling and benchmarking:
- Establish Baseline Metrics: Measure key performance indicators (KPIs) for your application before implementing virtual threads.
- Identify Performance Bottlenecks: Use profiling tools to pinpoint areas of your application that could benefit most from virtual threads.
- Iterative Testing: Implement virtual threads in stages, testing and measuring performance at each step.
- Stress Testing: Subject your application to high load scenarios to ensure it performs well under pressure.
2. Start Small, Scale Gradually
When it comes to implementing virtual threads, the tortoise approach often beats the hare. Starting small and scaling gradually allows you to identify and address issues early, before they become major problems.
A phased approach might look like this:
- Pilot Project: Implement virtual threads in a non-critical component of your application.
- Analyze and Adjust: Carefully monitor the performance and behavior of your pilot implementation, making adjustments as needed.
- Gradual Expansion: Slowly expand the use of virtual threads to other parts of your application, always monitoring and adjusting as you go.
- Full Implementation: Once you’re confident in your approach, roll out virtual threads across your entire application.
3. Monitor and Adjust
Implementing virtual threads isn’t a “set it and forget it” task. Continuous monitoring and adjustment are key to maintaining optimal performance.
Key areas to monitor include:
- Thread Creation and Termination Rates: Keep an eye on how many threads are being created and destroyed over time.
- Resource Utilization: Monitor CPU, memory, and I/O usage to ensure your application is using resources efficiently.
- Response Times: Track how virtual threads are impacting your application’s responsiveness.
- Error Rates: Monitor for any increase in errors or exceptions that might be related to concurrency issues.
4. Documentation and Knowledge Sharing
Documenting your journey with virtual threads is like leaving breadcrumbs for your future self (and your teammates). Good documentation can save hours of head-scratching down the line.
Tips for effective documentation:
- Record Design Decisions: Document why you chose certain approaches or configurations.
- Create Troubleshooting Guides: Document common issues and their solutions.
- Maintain a Best Practices Document: As you learn what works best, document these insights for future reference.
- Share Knowledge: Conduct knowledge-sharing sessions with your team to ensure everyone is on the same page.
5. Continuous Learning and Adaptation
The world of concurrent programming is ever-evolving, and staying ahead of the curve is crucial. Make continuous learning a part of your virtual thread journey.
Ways to stay updated:
- Follow Java Enhancement Proposals (JEPs): Keep an eye on upcoming changes and improvements to virtual threads.
- Participate in Community Discussions: Engage with other developers in forums, conferences, and online communities.
- Experiment with New Features: As new features and improvements are released, take the time to experiment with them in a safe environment.
- Read Research Papers: Stay informed about the latest academic research in concurrent programming.
Real-World Applications of Virtual Threads
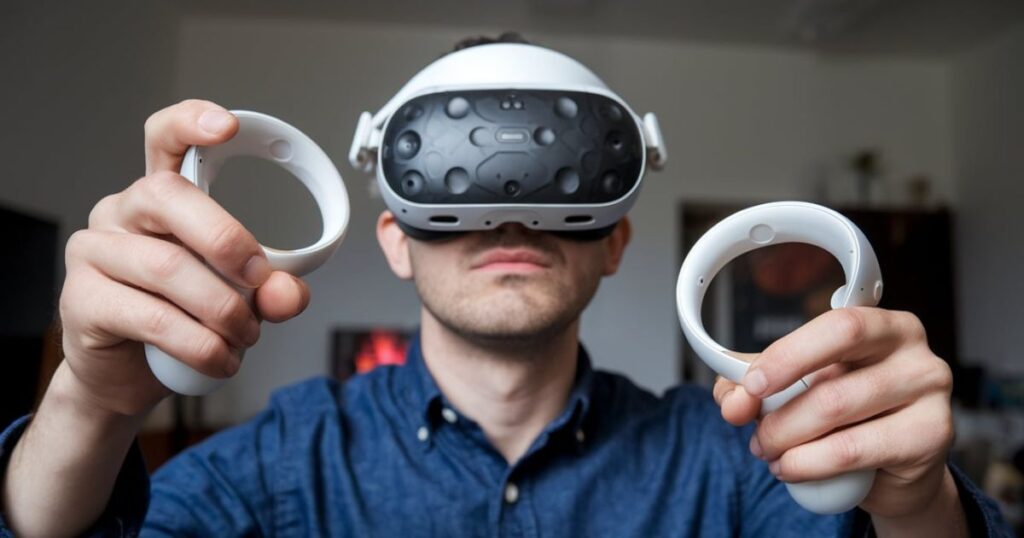
Virtual threads aren’t just theoretical constructs – they’re making a real impact in various domains. Let’s explore some real-world applications where virtual threads are shining.
1. Web Servers
Web servers are one of the most obvious beneficiaries of virtual threads. With the ability to handle thousands of concurrent connections efficiently, virtual threads are making web servers faster than Usain Bolt.
Benefits in web server applications:
- Increased Throughput: Handle more requests per second with the same hardware resources.
- Improved Responsiveness: Reduce latency by processing requests concurrently.
- Better Resource Utilization: Make more efficient use of system resources, especially in I/O-bound scenarios.
2. Financial Systems
In the world of finance, where milliseconds can mean millions, virtual threads are proving their worth. They’re particularly valuable in high-frequency trading systems where speed and concurrency are paramount.
Applications in financial systems:
- Real-time Data Processing: Handle massive streams of market data concurrently.
- Risk Analysis: Perform complex risk calculations across multiple scenarios simultaneously.
- Transaction Processing: Handle a high volume of concurrent transactions with low latency.
3. Gaming
The gaming industry is always pushing the boundaries of what’s possible with hardware, and virtual threads are opening up new possibilities for game developers.
- AI and NPC Behavior: Implement more complex and realistic AI behaviors by running multiple decision-making processes concurrently.
- Physics Simulations: Enhance the realism of in-game physics by running more detailed simulations in parallel.
- Multiplayer Networking: Improve the responsiveness and scalability of multiplayer game servers.
- Procedural Content Generation: Generate vast, detailed game worlds on-the-fly by leveraging concurrent processing.
4. Machine Learning
In the realm of machine learning, where processing vast amounts of data is the norm, virtual threads are proving to be a game-changer. They’re enabling researchers and developers to train models faster and process data more efficiently than ever before.
Applications in machine learning:
- Parallel Data Processing: Preprocess large datasets concurrently, significantly reducing preparation time.
- Distributed Training: Implement more efficient distributed training algorithms for neural networks.
- Real-time Prediction Services: Build high-performance prediction services capable of handling multiple requests simultaneously.
- Hyperparameter Tuning: Run multiple model configurations in parallel to find optimal hyperparameters more quickly.
Also Read: Unveiling the Genius: A Journey Through Brancusi’s Homeland from Bucharest
The Future of Virtual Threads
As we look to the future, it’s clear that virtual threads are poised to play an increasingly important role in the world of concurrent programming. Their ability to provide high levels of concurrency with relatively low overhead makes them an attractive option for a wide range of applications.
Some potential future developments to watch out for:
- Integration with Other JVM Languages: While currently a Java feature, we may see virtual threads being adopted by other JVM languages like Kotlin or Scala.
- Enhanced Debugging Tools: As virtual threads become more prevalent, we can expect to see more sophisticated debugging and profiling tools designed specifically for virtual thread-based applications.
- Optimizations in JVM: Future JVM versions may include further optimizations for virtual threads, potentially improving their performance even more.
- New Programming Patterns: As developers gain more experience with virtual threads, we’re likely to see new design patterns and best practices emerge.
Conclusion
In conclusion, virtual threads represent a paradigm shift in concurrent programming, offering developers a powerful tool to create high-performance, scalable applications. By embracing virtual threads and skillfully navigating their potential pitfalls, you can unlock new levels of efficiency and responsiveness in your Java applications. Remember to start small, monitor closely, and continuously adapt your approach as you gain experience.
The future of programming is undoubtedly concurrent, and virtual threads are at the forefront of this revolution. As you embark on your journey with virtual threads, stay curious, keep learning, and don’t be afraid to push boundaries. With virtual threads in your toolkit, you’re well-equipped to tackle the challenges of modern software development and create applications that truly stand out in today’s competitive landscape.